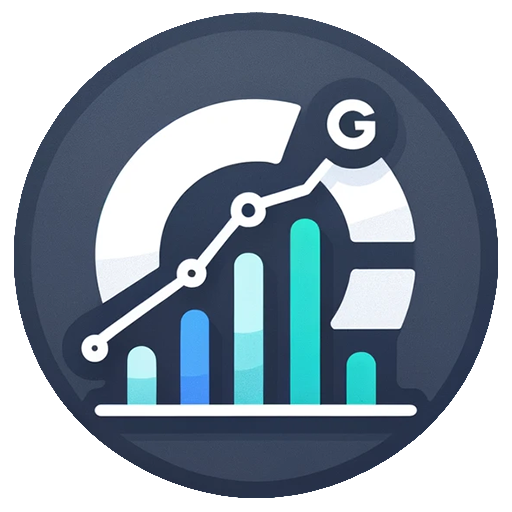
Go Benchmarks
Visual comparison of different Go benchmarks
Array vs Slice
A benchmark to compare the performance of arrays, pre-allocated slices and dynamic slices.
Implementations:
- Array
- Dynamic Slice
- Preallocated Slice
Concurrent Map Access
A benchmark to compare the performance of different concurrent map access implementations in Go.
Implementations:
- Mutex
- Sync
Counter
A benchmark to compare the performance of different counter implementations in Go.
Implementations:
- Atomic Pointer Counter
- Atomic Uint Counter
- Int Counter
- Int Counter With Mutex
Functions:
- get
- increment
Demo
A demo benchmark to preview all the functions of go-benchmarks and to validate them.
Implementations:
- Faster Over Time
- Faster With More CPU Cores
- Slower Over Time
fmt
A benchmark of different fmt functions, of the Go standard library.
Functions:
- println
- printf
Interface vs Direct Method Call
A benchmark to compare the performance of interface vs direct method calls in Go.
Implementations:
- Direct Method Call
- Interface Method Call
Sorting Algorithms
A benchmark to compare the performance of different sorting algorithms in Go.
Implementations:
- Bubble Sort
- Builtin Sort
- Insertion Sort
- Merge Sort
- Quick Sort
- Selection Sort
String Concatination
A benchmark to compare the performance of different string concatination implementations in Go.
Implementations:
- Append To Slice And Join
- Buffer
- Simple Append
- String Builder
Functions:
- read
- write
Synchronization Methods
A benchmark to compare the performance of channel and mutex synchronization in Go.
Implementations:
- Channel
- Mutex